
When traversing a tree, the recursion depth is limited by the tree depth. Reversing a linked list is not one of those. Recursion makes some algorithms really elegant and short, and it is the natural way to implement some algorithms, such as tree traversal. If I copy your list, then I will probably get a double delete error at runtime, but this is not guaranteed. Please provide a copy and move constructor and assignment operator. For example, you can provide a public iterator, const_iterator alias to basically pointers to the individual elements inside nodes and provide begin and end as functions. You can provide an iterator interface, so that your container works with the range for loop.

You can use in-class initializers for example for Node::next. Sometimes you use the implicit conversion to bool for pointers and sometimes not. You could provide a constructor taking an std::initializer_list, so you could specify the elements of the list directly on construction. Mark classes that one shouldn't inherit from with final.
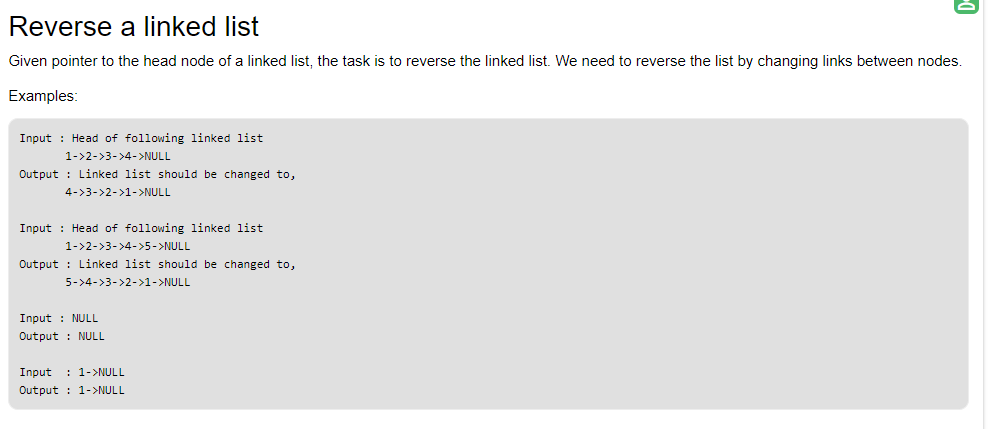
Mark functions that do not throw noexcept, if you compile with exceptions. That way, insertion is O(1) instead of O(n). It might be "better" to keep a pointer to the last node in your linked list. You should also be able to merge both functions. In LinkedList::recursiveReverse the first overload, you don't need to copy head because the other overload you're calling isn't modifying its arguments (no pass by reference). I won't claim it's more efficient, because any compiler worth its salt is going to optimize the call to std::strlen. Single characters should be in single quotes, not double quotes. Same goes for LinkedList::insert and so on. It should be data(std::move(value)) so that no copy is made.
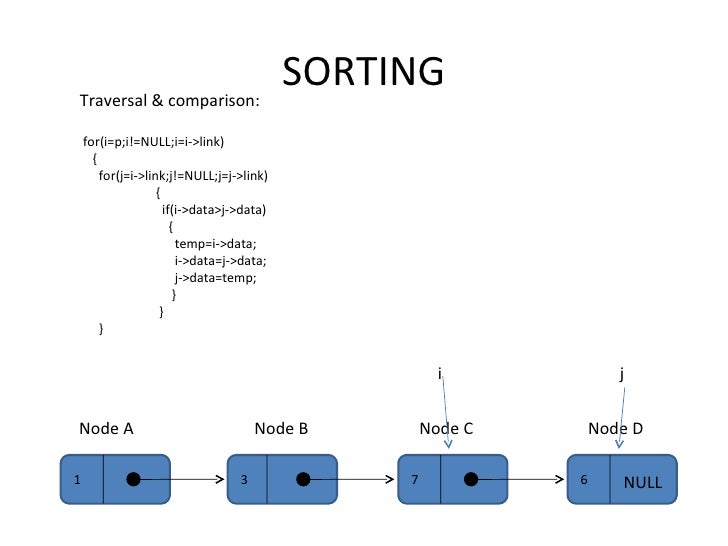
Node::Node unnecessarily copies value into data. You can replace this with a call to std::exchange: delete std::exchange(head, head->next) You have this in the destructor: tmp = head No warnings with very pedantic clang flags, good job! Node *remainingList = recursiveReverse(head) Node *secondElement = firstElement->next įirstElement->next = nullptr //unlink first node Node(T value) : data(value), next(nullptr)
READING LINKED LIST STACK BACKWARDS CODE
I want to optimise this code and improve it using advanced C++.
